API Access
Mona's API is based on Bearer Authorization tokens.
Retrieving the JWT token is possible by passing the clientId and the API secret to the authentication endpoint.
Generating clientId and secret
Both the clientId and secret are found in the Admin page under API Tokens.
To get a new clientId and secret click on "Add new token".
After clicking "Add new token", add the token description and which roles can use this token.
NOTE: The only relevant use-case today is to use the “admin” role for API access - but this will change as more functions will be exposed via API.
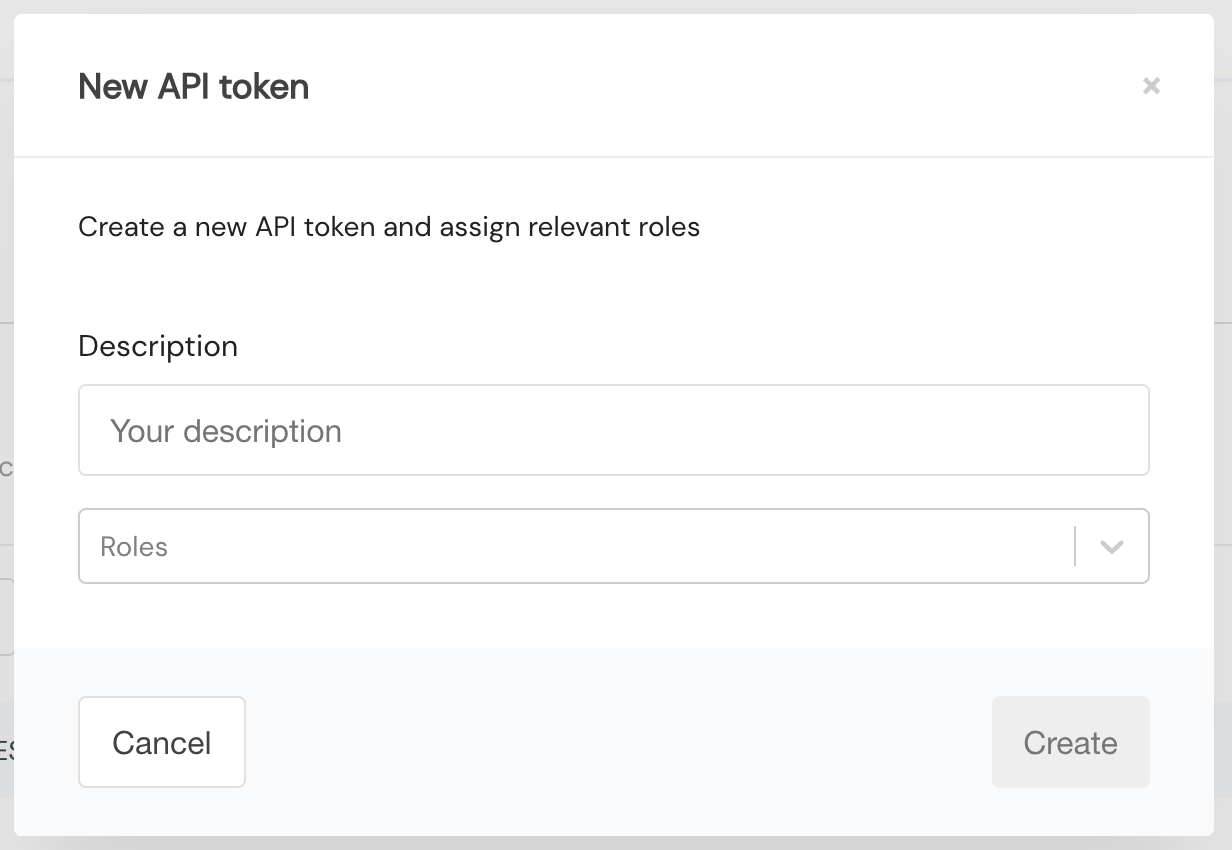
Once created you can see both clientId and secret in the pop-up window. Make sure to copy the secret to a safe place since this is the only time you’ll be able to see it on Mona.
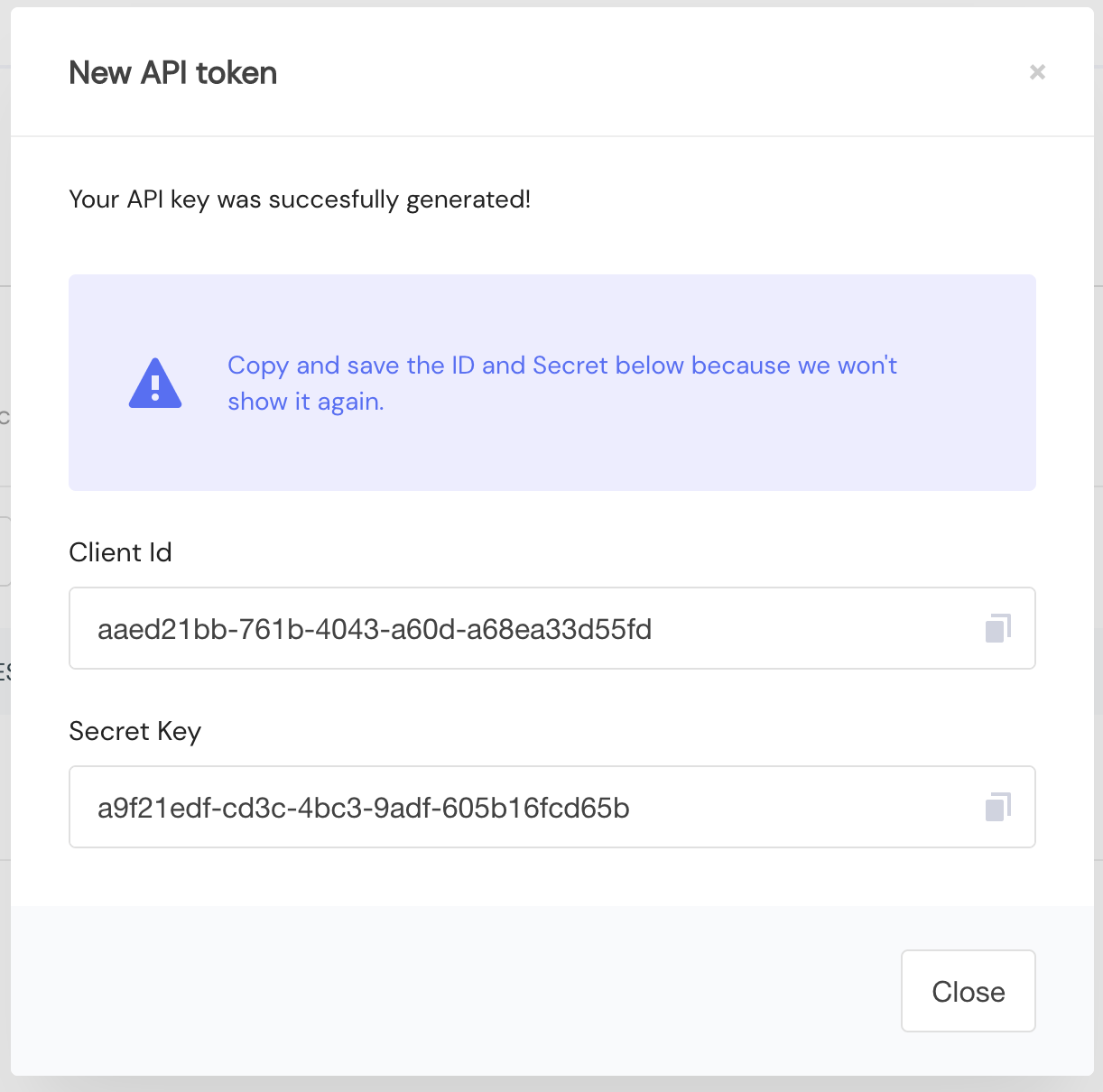
Generating a New JWT from Your ClientId and Secret
Now that you have a clientId and secret we can generate an API Token by sending an authentication request to the authentication endpoint with both the clientId and secret.
curl --location --request POST 'https://monalabs.frontegg.com/identity/resources/auth/v1/api-token' \
--header 'Content-Type: application/json' \
--data-raw '{
"clientId": "aaed21bb-761b-5053-a60d-a68ea33d55fd",
"secret": "a9f21edf-cd3c-4bc3-3adf-305b16fcd65b"
}'
import requests
url = "https://monalabs.frontegg.com/identity/resources/auth/v1/api-token"
headers = {
'Content-Type': 'application/json'
}
payload={
"clientId": "aaed21bb-761b-5053-a60d-a68ea33d55fd",
"secret": "a9f21edf-cd3c-4bc3-3adf-305b16fcd65b"
}
response = requests.request("POST", url, headers=headers, json=payload)
print(response.text)
The response for this request will be a JSON with expiration date, seconds until expiration, the access token and a refresh token.
{
"expires": "Thu, 08 Apr 2021 13:51:05 GMT",
"expiresIn": 14400,
"accessToken": "eyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiJhYWVkMjFiYi03NjFiLTQwNDMtYTYwZC1hNjhlYTMzZDU1ZmQiLCJ0ZW5hbnRJZCI6Im1vbmFfcHVibGljIiwicm9sZXMiOltdLCJwZXJtaXNzaW9ucyI6W10sIm1ldGFkYXRhIjp7fSwiY3JlYXRlZEJ5VXNlcklkIjoiMmM1MDUyNDQtZjNkNC00NThlLWI3MGUtNzExNWM5NTMyNGY4IiwidHlwZSI6InRlbmFudEFwaVRva2VuIiwiaWF0IjoxNjE3ODAzNDY1LCJleHAiOjE2MTc4ODk4NjUsImlzcyI6ImZyb250ZWdnIn0.QErTcxueqyHMVG-L_oR_IqS-7esvjHhVoPthpduxJ7PKRh2F_enR8FzaZT7LaX_fsFL9N4FGGzlS88pOEs_pYggPyrqzSIytfx4CdxLgvfsCSplTOh2CfmAt8IZ035uNuZyZowxIAyhbNpaQzt9ZzRcZW64AY_5ajPyNpSoArak",
"refreshToken": "fa5478a6-80fa-4c44-905d-1f55ccaa1e15"
}
Each token is valid for 4 hours. After the token expires it can be refreshed with the refreshToken.
Refreshing the Token
In order to refresh an access token a refresh request must be sent to the token refresh endpoint, with the refreshToken from the response of the new token generation.
curl --location --request POST 'https://monalabs.frontegg.com/identity/resources/auth/v1/api-token/token/refresh' \
--header 'Content-Type: application/json' \
--data-raw '{
"refreshToken": "fa5478a6-80fa-4c44-905d-1f55ccaa1e15"
}'
import requests
url = "https://monalabs.frontegg.com/identity/resources/auth/v1/api-token/token/refresh"
headers = {
'Content-Type': 'application/json'
}
payload={
"refreshToken": "fa5478a6-80fa-4c44-905d-1f55ccaa1e15"
}
response = requests.request("POST", url, headers=headers, json=payload)
print(response.text)
The response for this request will be the same as in the new token request.
Updated 12 months ago